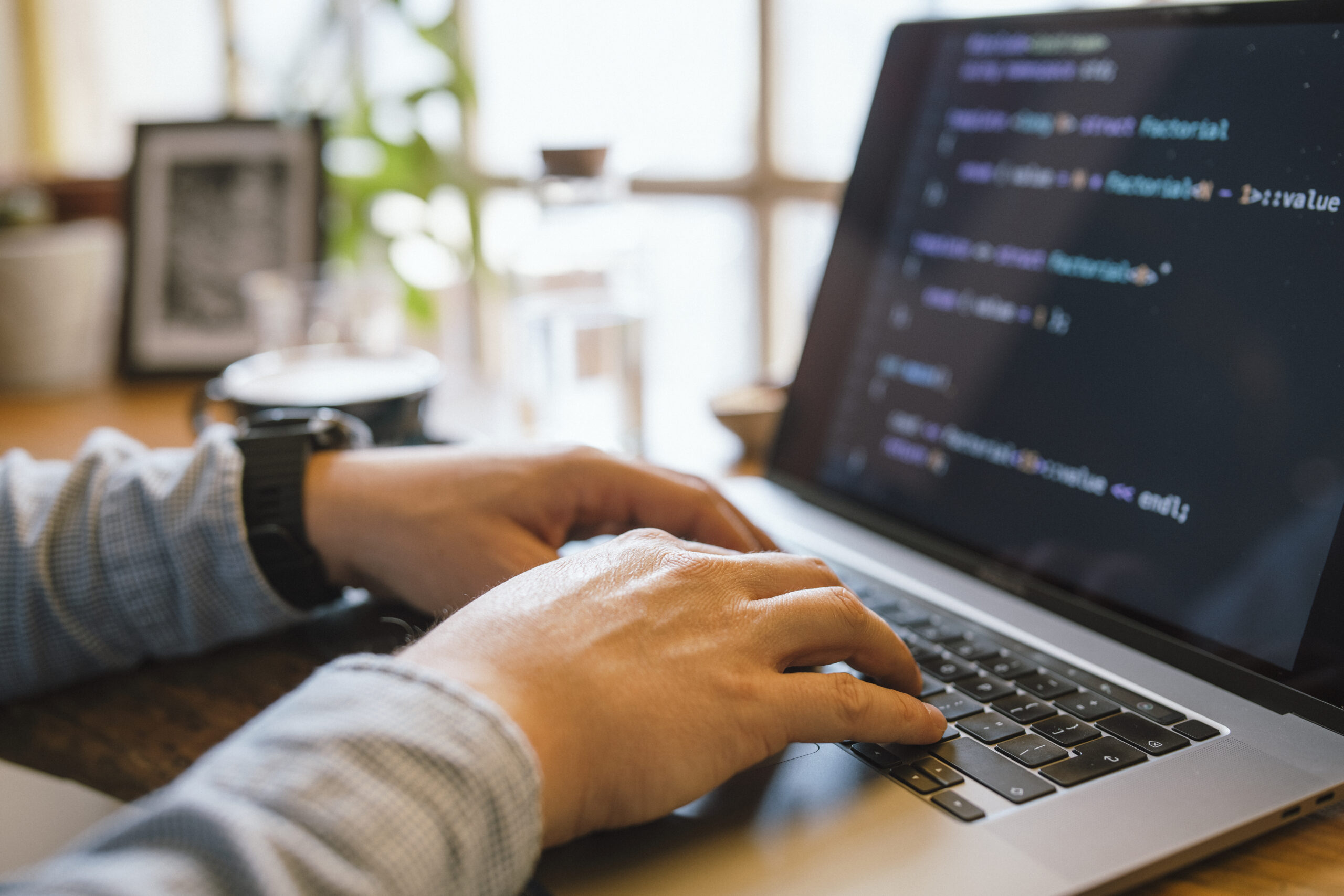
Debugging is Probably the most important — nevertheless generally missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to solve issues effectively. No matter whether you're a novice or even a seasoned developer, sharpening your debugging capabilities can save hours of frustration and dramatically improve your efficiency. Here are a number of methods to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
Among the list of quickest methods developers can elevate their debugging competencies is by mastering the instruments they use every single day. Though producing code is one particular Portion of improvement, knowing ways to connect with it proficiently for the duration of execution is equally vital. Modern-day advancement environments arrive equipped with highly effective debugging capabilities — but many builders only scratch the surface of what these applications can perform.
Get, for example, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications permit you to established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code within the fly. When used effectively, they let you notice precisely how your code behaves through execution, which can be invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They help you inspect the DOM, keep track of community requests, view true-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or technique-degree developers, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around operating processes and memory management. Understanding these instruments may have a steeper Understanding curve but pays off when debugging effectiveness challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, develop into comfortable with Edition Management devices like Git to understand code background, obtain the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications implies heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress atmosphere in order that when troubles come up, you’re not dropped at nighttime. The higher you recognize your applications, the greater time you could expend resolving the particular dilemma in lieu of fumbling as a result of the procedure.
Reproduce the condition
One of the more important — and infrequently neglected — ways in productive debugging is reproducing the situation. Ahead of jumping in to the code or making guesses, builders need to produce a reliable setting or situation exactly where the bug reliably seems. Devoid of reproducibility, correcting a bug gets a recreation of opportunity, normally resulting in wasted time and fragile code changes.
The first step in reproducing a challenge is collecting just as much context as is possible. Request questions like: What steps led to the issue? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've got, the less difficult it results in being to isolate the exact conditions underneath which the bug occurs.
When you finally’ve collected enough facts, attempt to recreate the condition in your local ecosystem. This could signify inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at producing automatic exams that replicate the sting cases or condition transitions associated. These tests not merely aid expose the situation but also avert regressions Down the road.
Occasionally, The problem may very well be setting-unique — it might come about only on sure operating techniques, browsers, or underneath individual configurations. Employing applications like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the problem isn’t merely a action — it’s a mindset. It demands persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you happen to be by now midway to correcting it. That has a reproducible state of affairs, You may use your debugging tools a lot more properly, examination likely fixes safely and securely, and converse additional Plainly with the team or users. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Go through and Realize the Error Messages
Error messages are often the most valuable clues a developer has when something goes Completely wrong. Instead of seeing them as frustrating interruptions, builders really should understand to deal with error messages as immediate communications within the process. They typically let you know precisely what happened, wherever it took place, and often even why it occurred — if you know how to interpret them.
Start out by looking through the message diligently As well as in complete. Several builders, particularly when under time tension, glance at the first line and straight away start off producing assumptions. But deeper from the error stack or logs may perhaps lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — browse and recognize them first.
Split the error down into areas. Is it a syntax error, a runtime exception, or simply a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These questions can information your investigation and level you towards the responsible code.
It’s also handy to know the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally comply with predictable patterns, and Understanding to acknowledge these can drastically accelerate your debugging system.
Some mistakes are obscure or generic, As well as in Individuals scenarios, it’s essential to examine the context where the mistake occurred. Examine linked log entries, input values, and recent improvements during the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized problems and provide hints about opportunity bugs.
Ultimately, error messages will not be your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, reduce debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most highly effective instruments inside of a developer’s debugging toolkit. When utilized efficiently, it provides actual-time insights into how an application behaves, assisting you realize what’s taking place under the hood with no need to pause execution or action from the code line by line.
A superb logging approach commences with being aware of what to log and at what degree. Frequent logging ranges consist of DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts for the duration of growth, Data for common gatherings (like profitable commence-ups), WARN for opportunity difficulties that don’t split the application, Mistake for genuine complications, and Deadly once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and decelerate your technique. Concentrate on key gatherings, state changes, enter/output values, and critical conclusion details within your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and function names, so it’s much easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting This system. They’re Specifically valuable in creation environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. Using a perfectly-believed-out logging technique, you can decrease the time it will require to spot troubles, gain deeper visibility into your programs, and Increase the overall maintainability and reliability of the code.
Imagine Like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently identify and repair bugs, developers have to solution the process just like a detective fixing a thriller. This mentality helps break down complicated concerns into manageable areas and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or effectiveness problems. Much like a detective surveys a crime scene, gather as much relevant info as you are able to with out jumping to conclusions. Use logs, test circumstances, and consumer reviews to piece with each other a clear photo of what’s occurring.
Following, kind hypotheses. Request oneself: What may very well be resulting in this habits? Have any adjustments not too long ago been produced to the codebase? Has this issue happened in advance of beneath comparable conditions? The objective is to slender down opportunities and discover possible culprits.
Then, test your theories systematically. Endeavor to recreate the challenge inside a controlled environment. When you suspect a particular function or ingredient, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcome lead you nearer to the read more truth.
Pay out shut focus to small aspects. Bugs typically hide inside the the very least anticipated places—similar to a missing semicolon, an off-by-one mistake, or perhaps a race affliction. Be comprehensive and affected individual, resisting the urge to patch The difficulty with no totally being familiar with it. Momentary fixes might disguise the real dilemma, just for it to resurface later.
Last of all, retain notes on what you experimented with and learned. Just as detectives log their investigations, documenting your debugging system can conserve time for long run problems and support Many others realize your reasoning.
By thinking like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in elaborate methods.
Generate Tests
Creating exams is among the best tips on how to improve your debugging expertise and Total progress performance. Checks not merely enable capture bugs early but will also function a security Web that offers you confidence when creating modifications in your codebase. A properly-analyzed software is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when an issue occurs.
Start with device checks, which deal with personal functions or modules. These little, isolated tests can rapidly reveal whether a specific bit of logic is Performing as predicted. Each time a examination fails, you promptly know wherever to seem, drastically lowering time spent debugging. Device assessments are Specifically helpful for catching regression bugs—problems that reappear after Beforehand remaining fastened.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These assistance be sure that a variety of elements of your software do the job jointly easily. They’re especially practical for catching bugs that arise in complicated units with a number of components or products and services interacting. If anything breaks, your tests can inform you which Section of the pipeline failed and underneath what circumstances.
Producing exams also forces you to definitely Believe critically regarding your code. To test a aspect effectively, you need to grasp its inputs, anticipated outputs, and edge conditions. This amount of being familiar with In a natural way potential customers to higher code composition and fewer bugs.
When debugging a concern, crafting a failing check that reproduces the bug is often a powerful initial step. After the take a look at fails consistently, it is possible to focus on fixing the bug and look at your exam pass when The problem is solved. This approach ensures that the exact same bug doesn’t return in the future.
Briefly, writing exams turns debugging from a discouraging guessing game into a structured and predictable method—serving to you capture more bugs, more quickly and a lot more reliably.
Choose Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Using breaks will help you reset your head, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You may perhaps get started overlooking noticeable glitches or misreading code you wrote just several hours previously. In this particular condition, your brain becomes less efficient at trouble-resolving. A brief stroll, a coffee break, or even switching to another endeavor for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of an issue when they've taken time and energy to disconnect, allowing their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of longer debugging classes. Sitting in front of a display screen, mentally stuck, is not only unproductive and also draining. Stepping away permits you to return with renewed Power in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline would be to established a timer—debug actively for 45–60 minutes, then take a five–10 moment break. Use that point to maneuver all around, extend, or do anything unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging In the long term.
In brief, getting breaks is not a sign of weak point—it’s a sensible method. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to increase as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing important in the event you take some time to mirror and assess what went Completely wrong.
Start by asking your self several essential thoughts once the bug is resolved: What brought on it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit testing, code evaluations, or logging? The answers usually reveal blind spots inside your workflow or comprehending and enable you to Construct more powerful coding practices relocating forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and Whatever you uncovered. With time, you’ll start to see patterns—recurring challenges or prevalent problems—which you could proactively prevent.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or A fast know-how-sharing session, supporting Other people steer clear of the very same challenge boosts group performance and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start off appreciating them as important aspects of your advancement journey. After all, many of the very best builders aren't those who publish perfect code, but individuals who continuously understand from their mistakes.
In the long run, each bug you correct provides a fresh layer on your skill set. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Bettering your debugging competencies requires time, follow, and tolerance — however the payoff is big. It will make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at That which you do.